Hi all, in this article I will explain how to upload a file by using Robot class. I want to keep this article as simple as possible. So, I will start with the scenario.
Test Scenario
- Open a demo website https://blueimp.github.io/jQuery-File-Upload/
- Click “Add files…” button.
- Use “uploadFileWithRobot” method to upload sw-test-academy.png image file under images folder.
- Check that image has been uploaded as shown below.
Test Code
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; import org.testng.Assert; import org.testng.annotations.AfterMethod; import org.testng.annotations.BeforeMethod; import org.testng.annotations.Test; import java.awt.*; import java.awt.datatransfer.Clipboard; import java.awt.datatransfer.StringSelection; import java.awt.event.KeyEvent; import java.util.concurrent.TimeUnit; public class RobotTest { public WebDriver driver; public WebDriverWait wait; private static String filePath = System.getProperty("user.dir") + "\\images\\sw-test-academy.png"; @BeforeMethod public void setup () { driver = new ChromeDriver(); driver.navigate().to("https://blueimp.github.io/jQuery-File-Upload/"); driver.manage().timeouts().pageLoadTimeout(15, TimeUnit.SECONDS); wait = new WebDriverWait(driver,10); driver.manage().window().maximize(); } @Test public void robotTest () throws InterruptedException { //Click Image Upload wait.until(ExpectedConditions.elementToBeClickable(By.cssSelector(".btn.btn-success.fileinput-button"))); driver.findElement(By.cssSelector(".btn.btn-success.fileinput-button")).click(); uploadFileWithRobot(filePath); Assert.assertTrue(wait.until(ExpectedConditions.visibilityOfElementLocated (By.cssSelector(".name"))).getText().equals("sw-test-academy.png")); //I added sleep to see the result with my eyes. If you want you can remove below line. Thread.sleep(2000); } @AfterMethod public void teardown () { driver.quit(); } //File upload by Robot Class public void uploadFileWithRobot (String imagePath) { StringSelection stringSelection = new StringSelection(imagePath); Clipboard clipboard = Toolkit.getDefaultToolkit().getSystemClipboard(); clipboard.setContents(stringSelection, null); Robot robot = null; try { robot = new Robot(); } catch (AWTException e) { e.printStackTrace(); } robot.delay(250); robot.keyPress(KeyEvent.VK_ENTER); robot.keyRelease(KeyEvent.VK_ENTER); robot.keyPress(KeyEvent.VK_CONTROL); robot.keyPress(KeyEvent.VK_V); robot.keyRelease(KeyEvent.VK_V); robot.keyRelease(KeyEvent.VK_CONTROL); robot.keyPress(KeyEvent.VK_ENTER); robot.delay(150); robot.keyRelease(KeyEvent.VK_ENTER); } }
GitHub Link: https://github.com/swtestacademy/RobotTest
[fusion_widget_area name=”avada-custom-sidebar-seleniumwidget” title_size=”” title_color=”” background_color=”” padding_top=”” padding_right=”” padding_bottom=”” padding_left=”” hide_on_mobile=”small-visibility,medium-visibility,large-visibility” class=”” id=””][/fusion_widget_area]
Thanks.
Onur
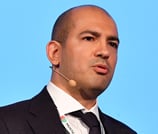
Onur Baskirt is a Software Engineering Leader with international experience in world-class companies. Now, he is a Software Engineering Lead at Emirates Airlines in Dubai.
I am having trouble getting this code to work on the grid. Have you tried this on the selenium grid?
No I did not try this on grid. What is the problem?
It does not recognize the window on the grid. The code works perfect locally
Thanks for the feedback. If Grid does not run on Windows based system yes it may cause a problem. Such as docker selenium or linux based system.
How to upload a file on mobile web application via Appium on chrome or safari or the same on chrome emulator.
Tried sendKeys and Robot class. Nothing works
Thanks
How to upload a file on mobile web application via Appium on Chrome or Safari browser or the same on chrome emulator via Selenium. Tried sendKeys() and Robot class. Nothing works Thanks
Eg website – https://smallpdf.com/pdf-converter
For Appium, I was able to push the file to the device folder using https://appiumpro.com/editions/2 but got stuck
For Chrome Emulator, I tried sendKeys() and robot class but it did not work as well. The file upload button element throws TimeOutException for waiting for its visibility
I found only this. I am sorry Rahul :(
https://stackoverflow.com/questions/51552445/how-to-upload-file-in-appium-for-mobile-app-automation
Unfortunately, there is no way to repeat Selenium javascript trick with sendKeys with native mobile application:
you need literally do everything via UI – open image selection, select image and etc.
The only thing that Appium can assist with is to upload files to device, so your device can be cleaned and populated only during the test.